Three js 绘制星空 电脑版发表于:2023/5/31 15:47 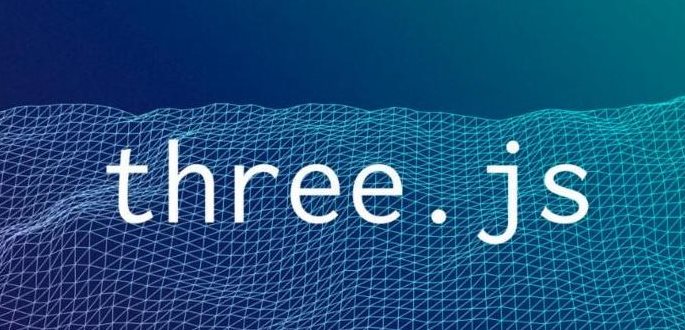 >#Three js 绘制星空 [TOC] 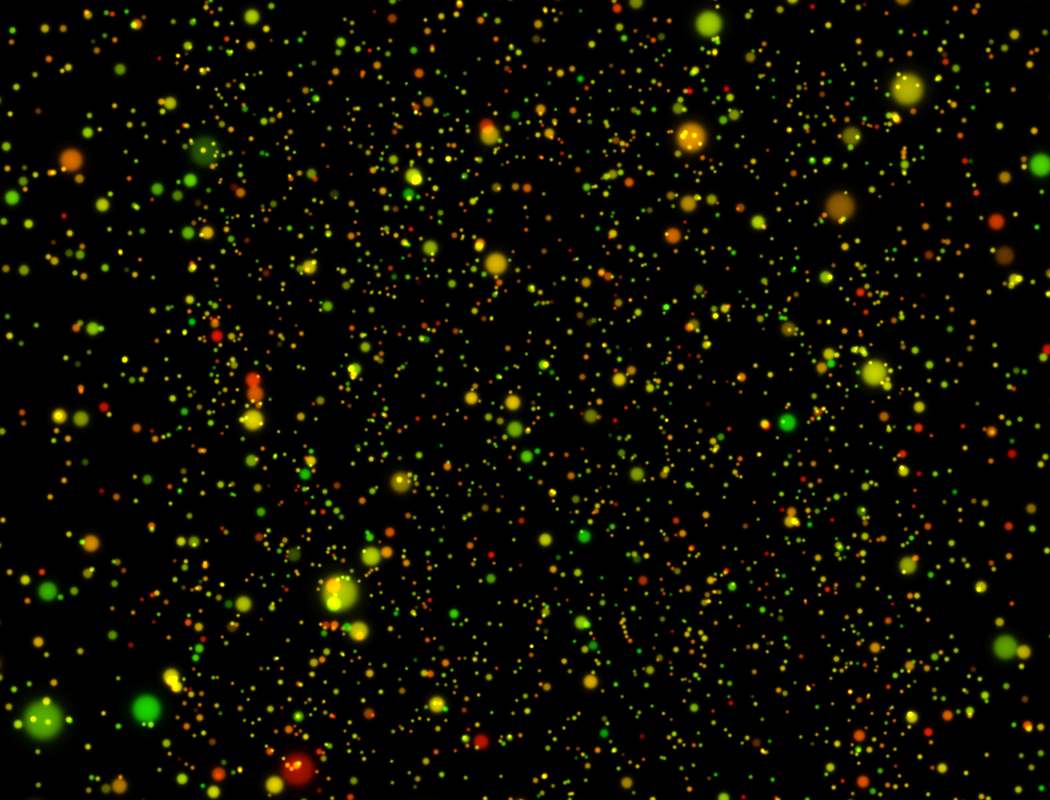 tn2>`package.json` ```json { "name": "01-three_basic", "version": "1.0.0", "description": "", "main": "index.js", "scripts": { "dev": "parcel src/index.html", "build": "parcel build src/index.html" }, "author": "", "license": "ISC", "devDependencies": { "parcel-bundler": "^1.12.5" }, "dependencies": { "dat.gui": "^0.7.9", "gsap": "^3.11.5", "three": "^0.152.2" } } ``` tn2>`index.html` ```json <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Document</title> <link rel="stylesheet" href="./assets/css/style.css"> </head> <body> <script src="./main/main.js" type="module"></script> </body> </html> ``` tn2>`main.js` ```javascript import * as THREE from 'three'; // 导入轨道控制器 import { OrbitControls } from 'three/examples/jsm/controls/OrbitControls' // 导入动画库 // import { gsap } from 'gsap'; // 导入dat.gui // import * as dat from 'dat.gui' // 创建平面 // 创建场景 const scene = new THREE.Scene() // 创建相机 // PerspectiveCamera 透视相机 const camera = new THREE.PerspectiveCamera( 75, window.innerWidth / window.innerHeight, 0.1, 1000) // 设置位置 // x,y,z camera.position.set(0, 0, 10); // 添加相机 scene.add(camera) // 添加辅助线 // const axesHelper = new THREE.AxesHelper( 5 ); // scene.add( axesHelper ); // 创建球几何体 // const sphereGeometry = new THREE.SphereGeometry(3,30,30); const sphereGeometry = new THREE.BufferGeometry(); const count = 5000; // 设置缓冲区数组 const positions = new Float32Array(count * 3); // 设置顶点 const colors = new Float32Array(count * 3) for (let i = 0; i < count * 3; i++) { positions[i] = (Math.random() - 0.5) * 30 colors[i] = Math.random(); } sphereGeometry.setAttribute('position',new THREE.BufferAttribute(positions,3)) sphereGeometry.setAttribute("color", new THREE.BufferAttribute(colors, 3)); // 设置点材质 const pointMaterial = new THREE.PointsMaterial() // 设置点大小 pointMaterial.size = 0.5; // 设置点颜色 pointMaterial.color.set(0xfff000) // 添加受光影响 pointMaterial.sizeAttenuation = true // 载入纹理 const textureLoader = new THREE.TextureLoader() const texture = textureLoader.load('./textures/particles/1.png') // 设置纹理 pointMaterial.map = texture; pointMaterial.alphaMap = texture; pointMaterial.transparent = true; pointMaterial.depthWrite = false; pointMaterial.blending = THREE.AdditiveBlending; // 启用顶点颜色 pointMaterial.vertexColors = true; // 创建点 const points = new THREE.Points(sphereGeometry, pointMaterial) // 场景添加点 scene.add( points ); // 初始化添加渲染器 const renderer = new THREE.WebGLRenderer(); // 设置渲染的尺寸大小 renderer.setSize(window.innerWidth,window.innerHeight) // 开启场景中的阴影贴图 renderer.shadowMap.enabled = true; renderer.useLegacyLights = true; // 将webgl渲染的canvas内容添加到body document.body.appendChild(renderer.domElement) // 创建控制器 const controls = new OrbitControls(camera, renderer.domElement) // 设置控制器阻力,让控制器更有真实效果,必须在你的动画循环里调用.update()。 controls.enableDamping = true; // 设置时钟 const clock = new THREE.Clock(); function render() { let time = clock.getElapsedTime(); // points.material.map.needsUpdate = true; // texture.needsUpdate = true // 对应设置控制器阻力 controls.update(); // 渲染render renderer.render(scene,camera); // 渲染下一帧的时候就会调用render函数 requestAnimationFrame( render ); } render() // 监听画面变化,更新渲染画面 window.addEventListener("resize", () => { // console.log("画面变化了"); // 更新摄像头 camera.aspect = window.innerWidth / window.innerHeight; // 更新摄像机的投影矩阵 camera.updateProjectionMatrix(); // 更新渲染器 renderer.setSize(window.innerWidth, window.innerHeight); // 设置渲染器的像素比 renderer.setPixelRatio(window.devicePixelRatio); }); ``` tn2>这里有个图。 