.netcore 3.1 MediatR:轻松实现命令查询职责分离模式(CQRS) 电脑版发表于:2020/10/26 17:50  >#.netcore 3.1 MediatR:轻松实现命令查询职责分离模式(CQRS) [TOC] <br/> >###中介者模式 tn>用一个**中介对象**封装一系列的对象交互,中介者使各对象不需要显示地相互作用,从而使耦合松散,而且可以独立地改变它们之间的交互。 使用**中介模式**,对象之间的交互将封装在中介对象中。对象不再直接相互交互(解耦),而是通过中介进行交互。这减少了对象之间的依赖性,从而减少了耦合。 中介者的**优点**与**缺点** >优点:中介者模式的优点就是减少类间的依赖,把原有的一对多的依赖变成了一对一的依赖,同事类只依赖中介者,减少了依赖,当然同时也降低了类间的耦合 缺点:中介者模式的缺点就是中介者会膨胀得很大,而且逻辑复杂,原本N个对象直接的相互依赖关系转换为中介者和同事类的依赖关系,同事类越多,中介者的逻辑就越复杂。 <br/> >###单播消息传输(Demo) tn>单播消息传输,也就是一对一的消息传递,一个消息对应一个消息处理。其通过 **IRequest** 来抽象单播消息,用 **IRequestHandler** 进行消息处理。 >创建项目 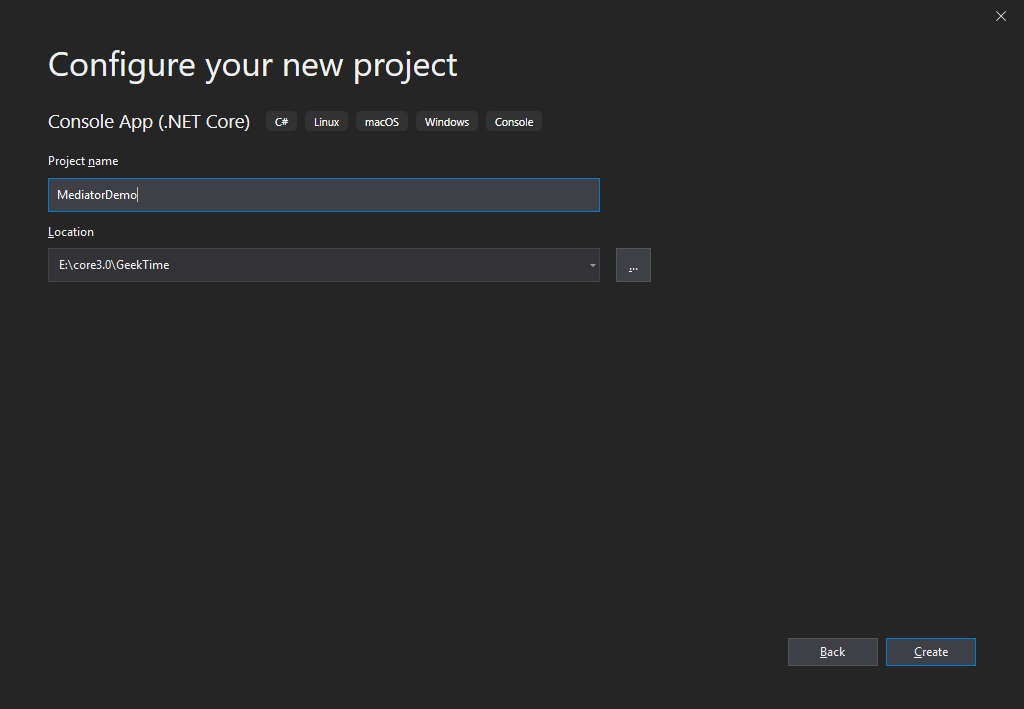 >添加下列nuget包到项目中 ```xml <ItemGroup> <PackageReference Include="MediatR" Version="9.0.0" /> <PackageReference Include="MediatR.Extensions.Microsoft.DependencyInjection" Version="9.0.0" /> <PackageReference Include="Microsoft.Extensions.DependencyInjection" Version="3.1.9" /> </ItemGroup> ``` 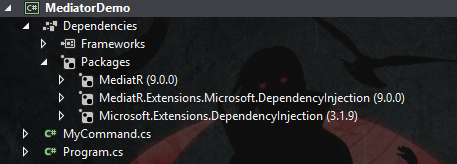 >添加 <font style="color:#2ecc71;font-weight:bold;">MyCommand.cs</font> ```csharp /// <summary> /// 创建 消息请求 /// </summary> internal class MyCommand : IRequest<long> { public string CommandName { get; set; } } /// <summary> /// 创建 消息处理 /// </summary> internal class MyCommandHandler : IRequestHandler<MyCommand, long> { public Task<long> Handle(MyCommand request, CancellationToken cancellationToken) { System.Console.WriteLine($"MyCommandHandler run command:{request.CommandName}"); return Task.FromResult(10L); } } ``` ><font style="color:#2ecc71;font-weight:bold;">Program.cs</font> ```csharp async static Task Main(string[] args) { var services = new ServiceCollection(); services.AddMediatR(typeof(Program).Assembly); var serviceProvider = services.BuildServiceProvider(); var mediator = serviceProvider.GetService<IMediator>(); await mediator.Send(new MyCommand { CommandName = "cmd01" }); Console.WriteLine("Hello World!"); } ``` >运行结果 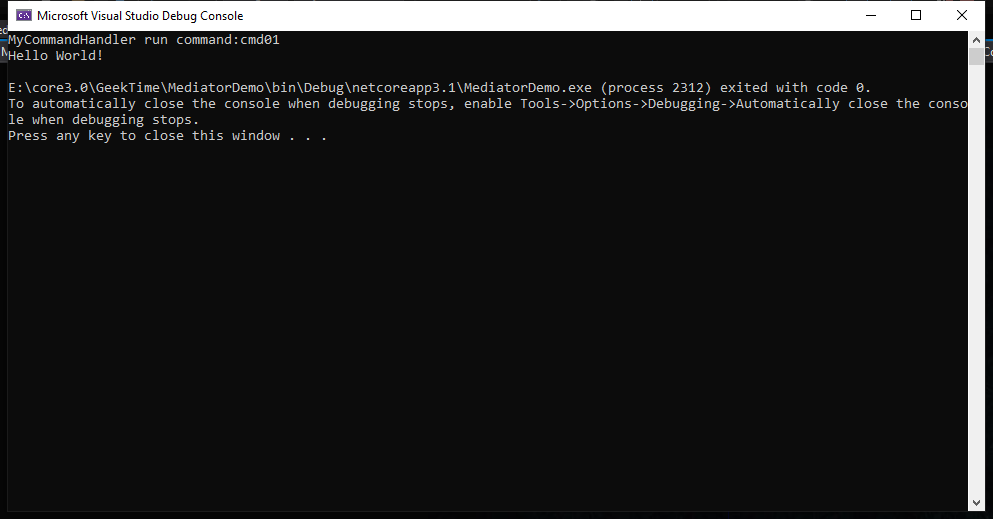 <br/> >###多播消息传输(Demo) tn>多播消息传输,也就是一对多的消息传递,一个消息对应多个消息处理。其通过**INotification**来抽象多播消息,对应的消息处理类型为**INotificationHandler**。 >添加 <font style="color:#2ecc71;font-weight:bold;">Ping.cs</font> ```csharp public class Ping : INotification { public string Name { get; set; } } //构建 消息处理器1 public class Pong1 : INotificationHandler<Ping> { public Task Handle(Ping notification, CancellationToken cancellationToken) { Console.WriteLine($"{notification.Name} Pong 1"); return Task.CompletedTask; } } //构建 消息处理器2 public class Pong2 : INotificationHandler<Ping> { public Task Handle(Ping notification, CancellationToken cancellationToken) { Console.WriteLine($"{notification.Name} Pong 2"); return Task.CompletedTask; } } ``` ><font style="color:#2ecc71;font-weight:bold;">Program.cs</font> ```csharp await mediator.Publish(new Ping() { Name="Start" }); ``` >运行结果 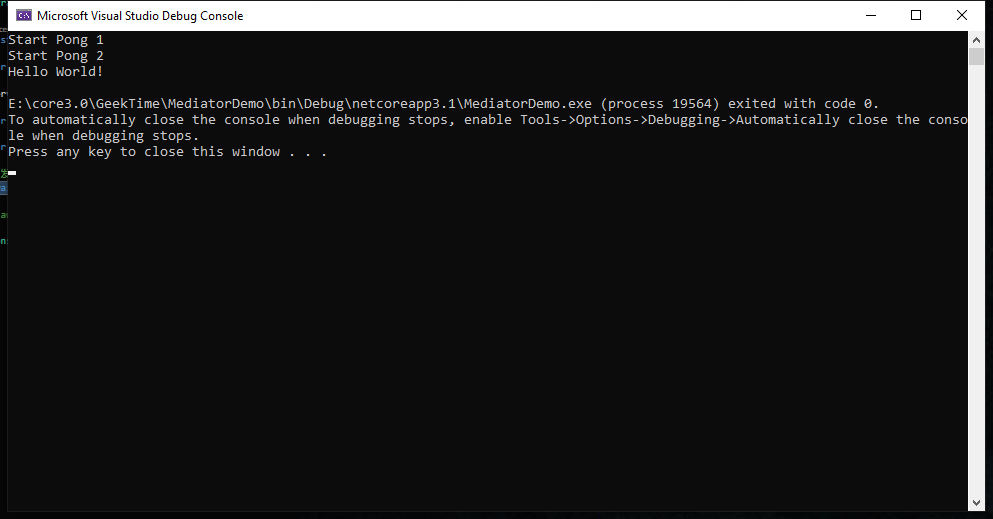