ASP.NET Core 作用域与对象释放行为 (基础)[学习笔记] 电脑版发表于:2020/5/9 13:36  >#ASP.NET Core 作用域与对象释放行为 (基础)[学习笔记] [TOC] <br/> 知识大纲 ------------ <br/> >作用域 - IServiceScope >实现IDisposable接口类型的释放 - DI只负责释放由其创建的对象实例 - DI在容器或子容器释放时,释放由其创建的对象实例 <br/> <br/> 代码准备 ------------ <br/> >###项目结构  >###项目中相关代码 <br/> ><font style="color:#2ecc71;font-weight:bold;">OrderService.cs</font> ```csharp public interface IOrderService { } public class DisposableOrderService : IOrderService, IDisposable { public void Dispose() { Console.WriteLine($"DisposableOrderService Disposed:{this.GetHashCode()}"); } } ``` ><font style="color:#2ecc71;font-weight:bold;">WeatherForecastController.cs</font> ```csharp [HttpGet] public int Get( [FromServices]IOrderService orderService, [FromServices]IOrderService orderService2, [FromServices]IHostApplicationLifetime hostApplicationLifetime, [FromQuery]bool stop = false ) { #region Console.WriteLine("=======1=========="+ " orderService :"+ orderService.GetHashCode()); using (IServiceScope scope = HttpContext.RequestServices.CreateScope()) { var service = scope.ServiceProvider.GetService<IOrderService>(); var service2 = scope.ServiceProvider.GetService<IOrderService>(); Console.WriteLine("=======2==========" + " service :" + service.GetHashCode()); Console.WriteLine("=======2==========" + " service2 :" + service2.GetHashCode()); } Console.WriteLine("=======3==========" + " orderService2 :" + orderService2.GetHashCode()); #endregion #region if (stop) { hostApplicationLifetime.StopApplication(); } #endregion Console.WriteLine("接口请求处理结束"); return 1; } ``` ><font style="color:#2ecc71;font-weight:bold;">Startup.cs</font> ```csharp public void ConfigureServices(IServiceCollection services) { services.AddTransient<IOrderService, DisposableOrderService>(); //services.AddSingleton<IOrderService>(p=>new DisposableOrderService()); //services.AddScoped<IOrderService, DisposableOrderService>(); services.AddControllers(); } // This method gets called by the runtime. Use this method to configure the HTTP request pipeline. public void Configure(IApplicationBuilder app, IWebHostEnvironment env, IHostApplicationLifetime hostApplicationLifetime) { //var s = app.ApplicationServices.GetService<IOrderService>(); //var s2 = app.ApplicationServices.GetService<IOrderService>(); if (env.IsDevelopment()) { app.UseDeveloperExceptionPage(); } app.UseRouting(); app.UseAuthorization(); app.UseEndpoints(endpoints => { endpoints.MapControllers(); }); } ``` <br/> Transient ------------ <br/> `services.AddTransient<IOrderService, DisposableOrderService>();` <br/> 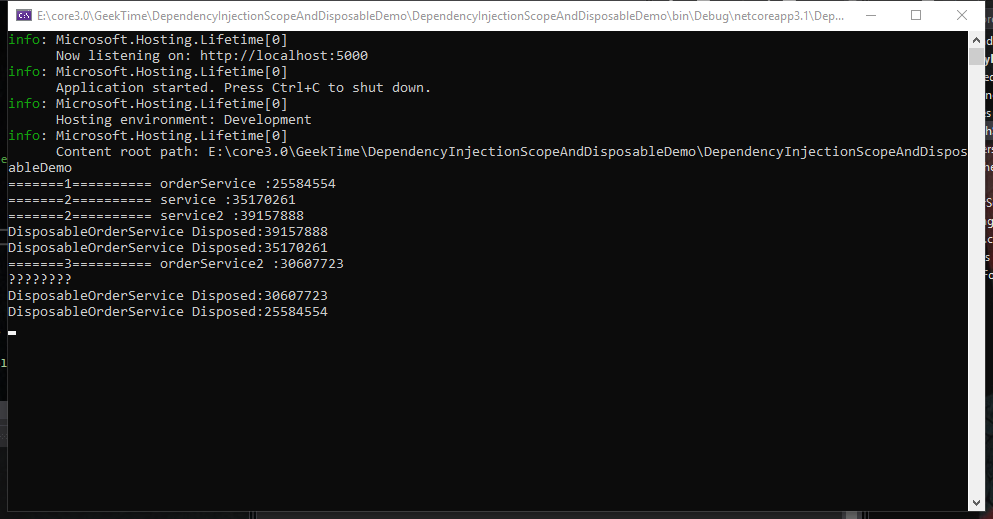 <br/> Singleton ------------ <br/> `services.AddSingleton<IOrderService>(p=>new DisposableOrderService());` <br/> 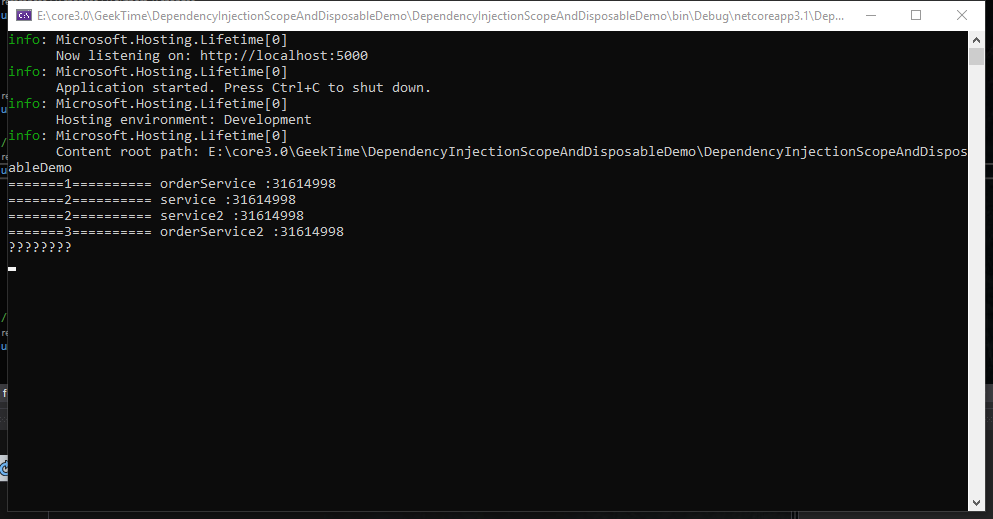 <br/> Scoped ------------ <br/> `services.AddScoped<IOrderService, DisposableOrderService>();` <br/> 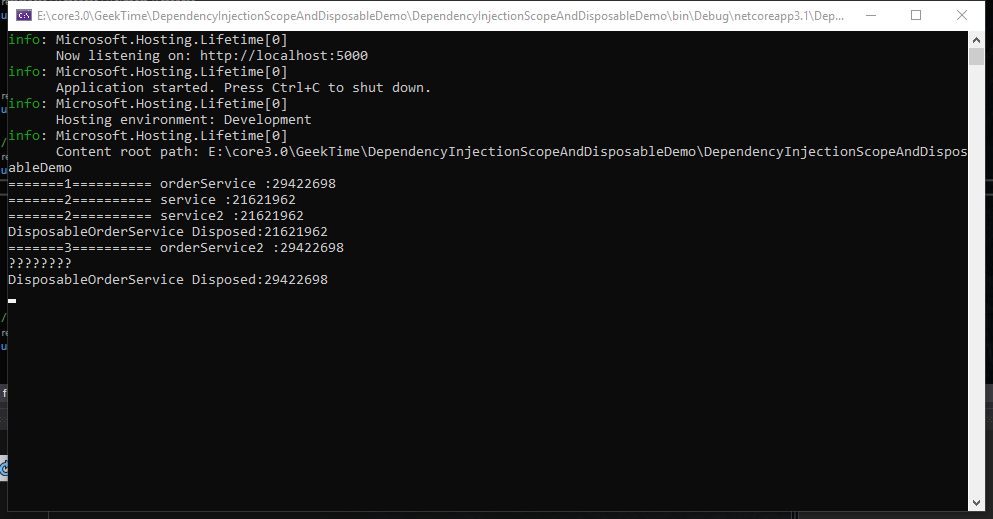 <br/> >从上述来看可以清晰的看到对象的释放情况,这里我们可以通过stop为true时再次去观察情况 | | | | ------------ | ------------ | | `hostApplicationLifetime.StopApplication();` | 关闭当前应用程序 | <br/> 项目地址 ------------ <br/> >GitHub: https://github.com/AiDaShi/GeekTimeLearning