android 绘制自定义控件,android绘制同心圆,android绘制小三角,android画虚线 电脑版发表于:2015/6/20 21:38 **一:实现同心圆加小三角指向效果** 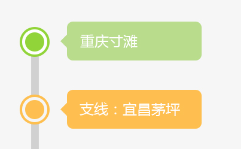 tn3>同心圆 ``` public class RingView extends View{ private Paint paint; private Context context; private int radius;//半径 private int color;//颜色值 public RingView(Context context) { // TODO Auto-generated constructor stub this(context, null); } public RingView(Context context, AttributeSet attrs) { super(context, attrs); // TODO Auto-generated constructor stub this.context = context; this.paint = new Paint(); this.paint.setAntiAlias(true); //消除锯齿 } @Override protected void onDraw(Canvas canvas) { // TODO Auto-generated method stub int center = getWidth()/2; //绘制内圆 paint.setStyle(Paint.Style.FILL); paint.setColor(Color.parseColor("#91d439")); canvas.drawCircle(center,center, 22, this.paint); //绘制外圆(空心) paint.setStyle(Paint.Style.STROKE); paint.setColor(Color.parseColor("#91d439")); paint.setStrokeWidth(6); canvas.drawCircle(center,center, 30, this.paint); super.onDraw(canvas); } public int getRadius() { return radius; } public void setRadius(int radius) { this.radius = radius; } public int getColor() { return color; } public void setColor(int color) { this.color = color; } } ``` 这里的getWidth可以根据控件设置的宽度来得到 tn4>小三角 ``` public class mytriangle extends View{ private Paint paint; private Context context; private int radius;//半径 private int color;//颜色值 public mytriangle(Context context) { // TODO Auto-generated constructor stub this(context, null); } public mytriangle(Context context, AttributeSet attrs) { super(context, attrs); // TODO Auto-generated constructor stub this.context = context; this.paint = new Paint(); this.paint.setAntiAlias(true); //消除锯齿 } @Override protected void onDraw(Canvas canvas) { float x= getX();//得到控件的所在位置 float y= getX(); paint.setStyle(Paint.Style.FILL); paint.setColor(Color.parseColor("#b9dc8c")); // canvas.drawText("画三角形:", 10, 200, paint); // 绘制这个三角形,你可以绘制任意多边形 Path path = new Path(); // y=(float) (y+15.5); path.moveTo(Dp2Px(context,x+10),Dp2Px(context,y));// 此点为多边形的起点 path.lineTo(Dp2Px(context,x), Dp2Px(context,y+7)); path.lineTo(Dp2Px(context,x+10), Dp2Px(context,y+14)); path.close(); // 使这些点构成封闭的多边形 canvas.drawPath(path,paint); super.onDraw(canvas); } public float Dp2Px(Context context, float dp) { final float scale = context.getResources().getDisplayMetrics().density; return (float) (dp * scale + 0.5f); } public int getRadius() { return radius; } public void setRadius(int radius) { this.radius = radius; } public int getColor() { return color; } public void setColor(int color) { this.color = color; } } ``` 布局文件中使用用全类名访问就可以了,还可以设置自动义属性, getx(),gety得到控件的所在的坐标位置 ``` int[] location = new int[2] ; getLocationOnScreen(location);//获取在整个屏幕内的绝对坐标包括通知栏 System.out.println("OnScreen x:"+location[0]+" y:"+location[1]); ``` 注意:不要以为获取到了这个位置设置下就可以让控件在具体的位置了,而是所在布局文件类型以,0,0开始,所有 不要去设置位置默认就在那个位置,设置了反而让控件不在本身正确的位置了 ``` <com.lc.mycontrol.RingView android:layout_width="100dp" android:layout_height="100dp" /> ``` **二:画虚线** 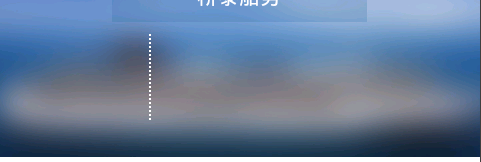 xml布局: ``` <com.lc.mycontrol.DashedLine android:id="@+id/dashedLine" android:layout_width="wrap_content" android:layout_marginLeft="50dp" android:layout_height="300dp" /> ``` view ``` public class DashedLine extends View{ private final String namespace = "http://com.smartmap.driverbook"; private float startX; private float startY; private float endX; private float endY; private Rect mRect; Context contextgb; public DashedLine(Context context, AttributeSet attrs) { super(context, attrs); contextgb = context; } @Override protected void onDraw(Canvas canvas) { int[] location = new int[2] ; //this.getLocationInWindow(location); //获取在当前窗口内的绝对坐标 //System.out.println("InWindow x:"+location[0]+" y:"+location[1]); this.getLocationOnScreen(location);//获取在整个屏幕内的绝对坐标 System.out.println("OnScreen x:"+location[0]+" y:"+location[1]); // TODO Auto-generated method stub super.onDraw(canvas); Paint paint = new Paint(); paint.setStyle(Paint.Style.STROKE); paint.setColor(Color.WHITE); Path path = new Path(); //location[0]获取到控件所在的x坐标,可以设置改控件位置 path.moveTo(5, Dp2Px(contextgb,10)); path.lineTo(5, Dp2Px(contextgb,56)); PathEffect effects = new DashPathEffect(new float[]{2,2,2,2},2); paint.setPathEffect(effects); paint.setStrokeWidth((float) 1.5); canvas.drawPath(path, paint); } public float Dp2Px(Context context, float dp) { final float scale = context.getResources().getDisplayMetrics().density; return (float) (dp * scale + 0.5f); } } ```